Lately I've been working on an extension for Google Chrome to read the last entry of the famous xkcd comic. At a certain point I stopped because I didn't understand how to dynamically resize the popup window of the Chrome extension. This required quite some research to understand what was going wrong, here is how I created the extension, which problem I had and how I solved it.
How I did it
The extension is very easy, it executes a GET request to the xkcd website, gets the content of the index page and returns it. What I used to...
Thursday, February 21, 2013
Friday, February 15, 2013
Posted by Masiar on 7:57 AM with No comments
I was printing some results for a chart while, after 10 minutes of executing the test, I realised I forgot to put the \n command after the number I wanted to print. I know the numbers I was printing were smaller than 10, so I came up with a useful command to append a new line after each character in a file:
sed 's/\(.\)/\1\n/g' -i filename
And that's it. As easy as that. I'm sorry for the lack of updates but lately I've been very bu...
Wednesday, February 13, 2013
Posted by Masiar on 7:11 AM with No comments
Today I was working while I was asked how to remove an event listener from an element which had an
click event. At first I didn't really remember how to do it, so I Googled a bit and I found this way:
document.getElementById("your_element_id").addEventListener('click', function(){
//do something
this.removeEventListener('click',arguments.callee,false);
}, false);
As you can see, all the work is done by addEventListener and removeEventListener functions. The first one takes as parameter the event (click in...
Tuesday, February 12, 2013
Posted by Masiar on 2:55 AM with No comments
I think everybody is familiar with the setInterval and the setTimeout functions. They create a timed event which will occur after a given timeout. setInterval creates an interval: after the given time it will repeat the same action over and over, while the setTimeout function will only execute the action once. Let's see a small example on how they work:
var timed_function = function(){
console.log("hello!");
}
var timeout = setInterval(timed_function, 1000);
The shown example will execute every second (1000 milliseconds)...
Friday, February 8, 2013
Posted by Masiar on 5:39 AM with No comments
In this final part I will show how I installed Node.JS on the Raspberry Pi on the Raspbian Wheezy OS.
First of all, open a terminal and execute the following commands:
cd /usr/src
sudo wget http://nodejs.org/dist/v0.8.16/node-v0.8.16.tar.gz
This will change the directory to /usr/src/ and download Node.JS version 0.8.16. I know this is not the last version, but I needed that because my project runs on it and it's not yet time to upgrade to the latest version (even though I'm pretty sure everything is going to be all right).
After the download...
Wednesday, February 6, 2013
Posted by Masiar on 11:08 AM with 1 comment

So after you plug everything (the power supply last), on the screen you should see Raspbian Wheezy booting. Lots of logs in the console and finally what looks like a BIOS screen:
If you don't then you will have the problem I had. In the past hours I struggled a lot because I couldn't see anything on the screen. I tried reinstalling the OS image on the SD (in at least three different ways) and plugging different devices but nothing happened. In...
Tuesday, February 5, 2013
Posted by Masiar on 6:37 AM with No comments
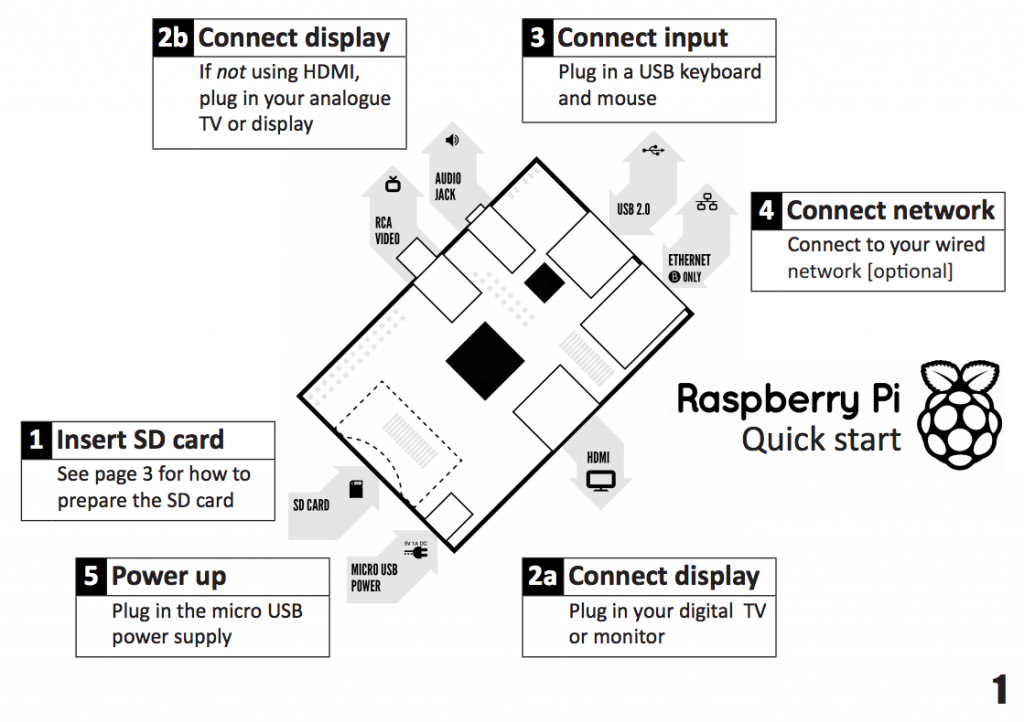
During these days I borrowed a Raspberry Pi. I would like to try to set up a Node.js server on it. I decided to write this post to show how I did the whole thing. Just to make things clear, I'm running on OSX.
So first of all once you have your Raspberry Pi, you should mount the OS image on the SD card. Some RPi comes with an SD card with everything setup. If that's the case, you are lucky and can skip this post. Otherwise, just connect the SD card...
Monday, February 4, 2013
Posted by Masiar on 9:31 AM with No comments
Some time ago I needed to measure the performance of the cores I was using on a machine while a Node.js server was running. I came up with different ideas, including writing a bash script that would measure it the hardcore way. I then realised I could use the os module that comes with Node and use the data it returns to measure it myself.
First of all, take a look at the specification of the method os.cpus() here. It shows how to call it and what is the return value. To measure the performance of the system, it is sufficient to take...
Sunday, February 3, 2013
Posted by Masiar on 10:38 AM with No comments
Some time ago I was talking with a second-year student after a C exam. He was pretty satisfied about the job done, but asked me a question about the ~ operator. At first I didn't quite remember, so I told him that was probably the bitwise not. Later I discovered I was right, so I took the opportunity to refresh my knowledge of the bitwise operations in JavaScript. The purpose of this article is to show which bitwise operations JavaScript offers.
First of all, we can only apply bitwise operations on numeric operands that have integer values. These...
Saturday, February 2, 2013
Posted by Masiar on 12:24 AM with No comments
Sometimes, even if it is considered bad habit, we need to check the class of an object in JavaScript. Classes may be defined by the programmer, but I will cover this topic later, for now let's just focus on classes defined by the interpreter. There are many, for example:
Object
String
Number
Date
Function
...
To check if an object is an instance of a specific class, JavaScript offers the instanceof method, which may be used in the following way:
var mynmbr = new Number(1);
var mystrng = new String("disasterjs!");
var myfunc = function(){
...
Friday, February 1, 2013
Posted by Masiar on 11:38 AM with No comments
Some time ago I had a problem with arrays and assignments. I was working with a distributed system in JavaScript; the root process sent some "work" to do to some subprocesses (workers) and those returned an array as result, which was modified inside the callback of the dispatching function.
The issue was that when I modified the array, I then assigned it to a variable and passed it to a function. The problem was that while I was working in the callback with this array, it got modified somehow. I later understood that the problem was due to the...
Posted by Masiar on 12:18 AM with 3 comments
One of the first issues I had with this blog was how to syntax-highlight code snippets. All I could find on the internet was garbage and old tutorials with the previous Blogger interface. Then I finally found this blog that proposed a working solution. Actually, it's the only solution that worked, so I'm suggesting it here, but I'm not entirely satisfied with the CSS that comes with it, so I might slightly modify it sooner or later.
If somebody knows a better solution, let me know in the comments, I'll be glad to take a look at ...
Subscribe to:
Posts (Atom)